Express
# 一. Express简介
Express 是一个极简而灵活的 node.js Web应用框架, 提供了一系列强大特性帮助你创建各种 Web 应用,和丰富的 HTTP 工具。Express中文官网 (opens new window)
为什么说Express是一个极简的框架
Express的核心只有两个部分
- 路由
- 中间件
Express提供了基本的路由处理和中间件功能, 几乎所有功能的实现都由一个个独立的中间件完成
# 1 路由
路由可以认为是一种找到数据的路径, 或者说是URL+处理函数
- 通过URL来区分不同的资源(页面或数据)
- 通过处理函数来返回资源(页面或数据)
前端通过URL请求数据.
后端通过不同的路由, 调用对应的方法, 返回指定的数据
# 2 中间件
顾名思义, 中间件就是在什么的中间
在请求和响应中间的处理程序
有时候从请求到响应的业务比较复杂, 将这些复杂的业务拆开成一个个功能独立的函数, 就是中间件
对于处理请求来说,在响应发出之前,可以在请求和响应之间做一些操作,并且可以将这个处理结果传递给下一个函数继续处理
express 中间件函数,帮助拆解主程序的业务逻辑,
并且每一个的中间件函数处理的结果都会传递给下一个中间件函数。
就好比工厂里流水线工人清洗一个箱子:
第一个人清洗侧面,第二个人清洗底面,第三个人清洗顶面,。。。
这条流水线结束后,箱子也就清洗干净了
各做各的,不相互影响,又彼此协作
2
3
4
5
6
# 二. 安装及使用
express也是一个node的包, 可以npm来安装
# 1 安装
npm i express
# 2 使用
步骤
- 导入express包
- 实例化对象
- 编写路由(中间件)
- 监听端口
示例
// 1. 引入express包
const express = require('express')
// 2. 实例化对象
const app = express()
// 3. 编写路由
app.get('/', function(req, res) {
res.send('hello world')
})
// 4. 监听端口
app.listen(3000)
2
3
4
5
6
7
8
9
10
# 三. 路由
路由模块由三部分组成
请求方式
URL
处理函数
路由模块主要方法是 app.METHOD (opens new window)
# 1 请求方式
请求方式就是HTTP协议的请求方式, 常见的有
- get: 对应
app.get()
- post: 对应
app.post()
- put: 对应
app.put()
- delete: 对应
app.delete()
# 2 URL
URL的写法
// 第一种, 不带参数
app.get('/users', function(req, res) {
res.send('hello world')
})
// 第二种, 带参数
app.get('/users/:id', function(req, res) {
res.send('hello world')
})
// 第三种, 正则表达式, 以html结尾
app.get(/.html$/, function(req, res) {
res.send('hello world')
})
2
3
4
5
6
7
8
9
10
11
12
# 3 处理函数
语法
function(req, res, next) {
// todo
}
2
3
在处理函数中, 有两个形参
- req(请求对象)
- res(响应对象)
# 1) 请求对象
请求对象包含了一次请求中的所有数据(http请求头, 请求参数...)
示例
app.get('/', function (req, res) {
console.log(req)
})
2
3
# 2) 获取请求参数
语法
// get请求
req.query.参数名
2
示例: get请求
// 如果url是: /users/1, 通过params获取
app.get('/users/:id', function(req, res) {
res.send(req.params.id)
})
// 如果url是: /users?id=1, 通过query获取
app.get('/users', function(req, res) {
res.send(req.query.id)
})
2
3
4
5
6
7
8
9
示例: post请求
// 1. 导入express包
const express = require('express')
// 2. 实例化对象
const app = express() // app是一个对象
// 3. 编写路由(中间件)
app.post('/', function(req, res) {
let postData = ''
req.on('data', data => postData += data)
req.on('end', () => {
res.send(JSON.parse(postData))
})
})
// 4. 监听端口
app.listen(3000, function () {
console.log('server is running on http://localhost:3000')
})
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
根据id查找对应的数据并返回
编写post.http
POST http://localhost:3000/
Content-Type: application/json
{
"name": "xiaoming"
}
2
3
4
5
6
# 3) 响应对象
响应对象用于向客户端返回数据, 在处理函数中需要调用以返回数据
常用的有两个
- res.send(): 返回各种类型
- res.json(): 返回json格式的数据
# 四. 中间件
# 1 中间件类型
# 2 应用级中间件
在Express中, 使用app.use
或者app.METHOD
注册的中间件叫做应用级中间件
中间件就是一个函数
app.use('path', function (req, res, next) {
next()
})
2
3
注意
在中间件中需要通过调用next()执行下一个中间件
如果不执行next(), 也没有调用send(). 这次请求将会被挂起
示例一
// 不写第一个参数, 给所有访问都注册了一个中间件
app.use(function (req, res, next) {
console.log('Time:', Date.now())
next()
})
2
3
4
5
示例二
app.use('/user/:id', function (req, res, next) {
console.log('Request Type:', req.method)
next()
})
app.get('/user/:id', function (req, res, next) {
res.send('USER')
})
2
3
4
5
6
7
8
示例三
可以同时注册多个中间件函数
app.use('/user/:id', function (req, res, next) {
console.log('Request Type:', req.method)
next()
}, function (req, res, next) {
console.log('Request Params:', req.params.id)
next()
})
app.get('/user/:id', function (req, res, next) {
res.send('USER')
})
2
3
4
5
6
7
8
9
10
11
示例四
app.use除了注册函数做为中间件外, 还可注册一个express.Router()
对象
const router = express.Router()
app.use('/user/:id', router)
2
# 3 路由级中间件
express.Router()
对象也可以注册中间件.
使用router.use
或者router.METHOD
注册的中间件叫做路由级中间件
var app = express()
var router = express.Router()
router.use(function (req, res, next) {
console.log('Time:', Date.now())
next()
})
router.get('/users/', function(req, res) {
res.send('hello')
})
2
3
4
5
6
7
8
9
10
路由级中间件的应用
当路由很多的时候, 如果全部写在app入口会使用文件过大, 不好维护. 可以把不同的路由拆分成多个模块
app.js
const express = require('express')
const userRouter = require('./routes/users.js')
const app = express()
app.use(function (req, res, next) {
console.log(req.url)
next()
})
// 加载路由
app.use('/users', userRouter)
app.listen(3000)
2
3
4
5
6
7
8
9
10
11
12
routers/users.js
const express = require('express')
const router = express.Router()
router.get('/', function(req, res) {
// 返回所有用户信息
const data = [
{id:1, username: 'xiaoming', age: 20},
{id:2, username: 'xiaomei', age: 18},
{id:3, username: 'xiaopang', age: 1},
]
res.json(data)
})
router.get('/:id', function(req, res) {
// 返回所有用户信息
const user = {id:1, username: 'xiaoming', age: 20}
res.json(user)
})
module.exports = router
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
# 五. 数据库操作
# 1 安装mysql库
npm i mysql
# 2 入门案例
操作数据库, 就是模拟客户端. 向MySQL的服务端发送SQL语句. 基本步骤如下:
- 引入mysql包
- 创建数据库连接
- 连接数据库
- 执行SQL查询
- 关闭连接
示例
// 1. 引入mysql包
const mysql = require('mysql')
// 2. 创建数据库连接
const con = mysql.createConnection({
host: 'localhost',
user: 'root',
password: '123456',
database: 'db'
})
// 3. 连接数据库
con.connect()
// 4. 执行查询
con.query('select * from student', function (err, res) {
if (err) throw err
console.log(res)
})
// 5. 关闭连接
con.end()
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
# 3 封装mysql
如果觉得有帮助, 可以微信扫码, 请杰哥喝杯咖啡~
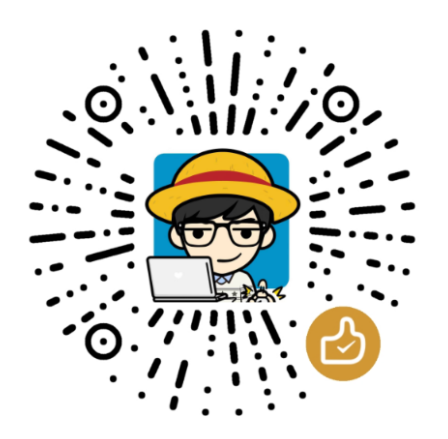